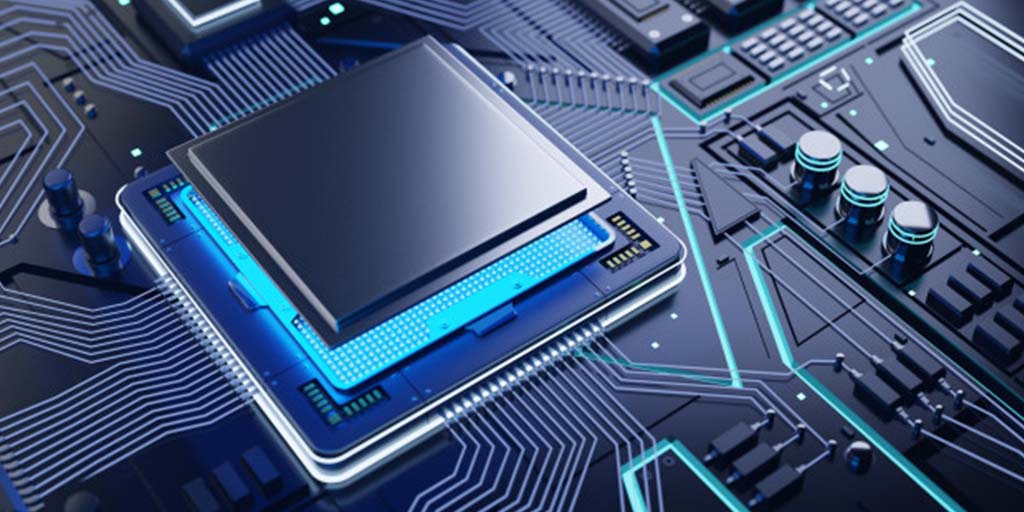
17 Jul Firebase controlled LED using Node MCU
What is Firebase??
The Firebase Realtime Database is cloud-hosted where the data is saved as JSON and contemporized in real-time with all the connected clients.
1. Open “firebase.google.com”
2. If you have a Gmail id, you don’t have to Sign Up for firebase. If you don’t have a Gmail id, Sign Up for firebase and proceed to the next step.
3. Click on “Go to Console” from the top right corner.

4. Select “Add project”.

5. Type your Project Name.
6. Accept the terms and conditions, create a new project, and select “Continue”.
7. Click on “Project Settings” from the settings option.

8. Now go to “Service Accounts”.

9. You will see “Firebase admin SDK” and “Database Secrets” options.
10. Go to “Database Secrets”.
11. Hover the cursor over your project name. The option “Show” will appear on the right side of your project.
12. Click on “Show” and the secret key generated for your project appears.

13. Copy and save the secret key in notepad. This is your “FIREBASE_AUTH” string, written in the Arduino program.
14. Now, go to “Database” from the left control bar.
15. Scroll down to select “Create Database”.

16. Choose “Enable” from “Start in test mode”.
17. You will see this right above the database:

18. Copy “your_project_name.firebaseio.com” without https and slash. Save this also to notepad along with the secret key.
19. This is your “FIREBASE_HOST” string, written in the Arduino program.
Upload the sketch when the “FIREBASE_AUTH” and “FIREBASE_HOST” are added in Arduino program. Now both the sections are set up and the complete Arduino Program is available.
BOM:
1. NodeMcu
2. Neopixel
Software:
1. Arduino IDE
2. Firebase service
Interfacing

Source code:
#include “FirebaseESP8266.h” | |
#include <ESP8266WiFi.h> | |
#include <Adafruit_NeoPixel.h> | |
#define PIN 5 | |
#define NUM_LEDS 1 | |
const char* ssid = “XXXXXX”; | |
const char* password = “XXXXXXX”; | |
FirebaseData firebaseData; | |
Adafruit_NeoPixel leds(NUM_LEDS, PIN, NEO_GRB + NEO_KHZ800); | |
// Current color values | |
int redValue = 0; | |
int greenValue = 0; | |
int blueValue = 0; | |
void setup() { | |
Serial.begin(9600); | |
connectWifi(); | |
leds.begin(); | |
Firebase.begin(“XXXXXXXXXXXXXXXXXXX “XXXXXXXXXXXXXXXXXXXXXXXXXX”); | |
} | |
void loop() { | |
if (Firebase.getInt(firebaseData, “/red”)) { | |
if (firebaseData.dataType() == “int”) { | |
int val = firebaseData.intData(); | |
if (val != redValue) { | |
redValue = val; | |
setLedColor(); | |
} | |
} | |
} | |
if (Firebase.getInt(firebaseData, “/green”)) { | |
if (firebaseData.dataType() == “int”) { | |
int val = firebaseData.intData(); | |
if (val != greenValue) { | |
greenValue = val; | |
setLedColor(); | |
} | |
} | |
} | |
if (Firebase.getInt(firebaseData, “/blue”)) { | |
if (firebaseData.dataType() == “int”) { | |
int val = firebaseData.intData(); | |
if (val != blueValue) { | |
blueValue = val; | |
setLedColor(); | |
} | |
} | |
} | |
} | |
void connectWifi() { | |
// Let us connect to WiFi | |
WiFi.begin(ssid, password); | |
while (WiFi.status() != WL_CONNECTED) { | |
delay(500); | |
Serial.print(“.”); | |
} | |
Serial.println(“…….”); | |
Serial.println(“WiFi Connected….IP Address:”); | |
Serial.println(WiFi.localIP()); | |
} | |
void setLedColor() { | |
for (int i=0; i < NUM_LEDS; i++) | |
leds.setPixelColor(i, leds.Color(redValue, greenValue, blueValue)); | |
leds.show(); | |
} |
view rawFirebase.ino hosted with ❤ by GitHub
Explanation
1) From include library function in Arduino and WIFI Library, add FirebaseESP8266 library. Use Neopixel to change the LED color.
- #include “FirebaseESP8266.h”
- #include <ESP8266WiFi.h>
- #include <Adafruit_Sensor.h>
2) Setup wifi connection using connectWifi().
- void connectWifi() {
- // Let us connect to WiFi
- WiFi.begin(ssid, password);
- while (WiFi.status() != WL_CONNECTED) {
- delay(500);
- Serial.print(“.”);
- }
- Serial.println(“…….”);
- Serial.println(“WiFi Connected….IP Address:”);
- Serial.println(WiFi.localIP());
- }
3) Setup function.
- Firebase.begin(“XXXXXXXXXXXXXXXXXXX “XXXXXXXXXXXXXXXXXXXXXXXXXX”);
To connect with firebase, use firebase host and Auth token.
4) Loop function checks the status of LED value and calls the function setLedColor.
- if (Firebase.getInt(firebaseData, “/red”)) {
- if (firebaseData.dataType() == “int”) {
- int val = firebaseData.intData();
- if (val != redValue) {
- redValue = val;
- setLedColor();
- }
5) The function setLedColor sets the neopixel color accordingly.
- void setLedColor() {
- for (int i=0; i < NUM_LEDS; i++)
- leds.setPixelColor(i, leds.Color(redValue, greenValue, blueValue));
- leds.show();
- }
If you still face issues in this topic, do not hesitate to post your queries in the Comment Box below. Also, for more such informative topics, follow us on 👍 Social Networks😉